When people first start using the Java Service Wrapper, they are usually interested in how to integrate their application with the Java Service Wrapper to run as a Service or to have their application monitored. Once an application is setup and integrated with other systems, the question of how to shut down often comes up.
There are a number of reasons why you would want to shut down an application in different ways. When a shutdown needs to be controlled by another application or system, for example, pressing CTRL-C is not an option.
We have been asked many times about the options available for controlling the shutdown process of the Wrapper, and decided that it was a good idea to collect them all in one place.
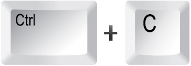
Solution |
As with many aspects of the Java Service Wrapper, it has been designed to be as flexible as possible to make integration with most Java applications possible. Over the years, we have built in several ways of controlling the Wrapper and its JVM to meet the needs of a wide variety of customers. Please take a look over the examples below to find which method (or methods) works best for you. The first few are most likely obvious, but some may be new even to experienced Wrapper users. |
Technical Background |
||||||||||||||||
Before getting into the different methods of shutting down the JVM, we will cover a few closely related topics that you need to understand or at least be aware of when thinking about shutting down your application.
|
Technical Overview |
||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Now that the main issues around application shutdown are understood, let's get into the options for shutting down an application.
|
Reference: Shutdown |
The Java Service Wrapper provides a full set of configuration properties that allows you to make the Wrapper meet your exact needs. Please take a look at the documentation for the individual properties to see all of the possibilities beyond the examples shown above.
|