On Windows systems, Java applications are run in a command prompt. This requires a user account to be logged on to the system at all times, and a command prompt to be open and running on the desktop.
There are several drawbacks to this ranging from security, to system performance, to simply having the risk of a user pressing the wrong key on the command prompt and killing the Java application.
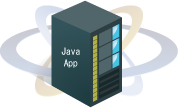
- What is a Service?
- Simple HelloWorldServer Java Class
- Wrapping the HelloWorldServer class
- Running the HelloWorldServer in a Console
- Simulate a Crash
- Running the HelloWorldServer as a Service
What is a Service? |
Windows has the ability to run applications in the background as a Service to solve these problems. Services are launched on system startup and do not require that a user be logged in. This greatly increases security and overall stability of the system because it is not possible for a user to stop, start, or otherwise tamper with them unless they are an administrator. The problem is that Java on its own can not be run as a service. Many simple tools like the Windows sc command can be used to run Java as a service, but the user doing something as simple as logging off of the machine will cause Java to shutdown. The Java Service Wrapper not only makes it possible, but makes it easy to run any Java application as a Windows Service. It also adds advanced failover, error recovery, and analysis features to make sure that your application has the maximum possible uptime. |
Simple HelloWorldServer Java Class |
||||||||||||||||||||||||
For this example, we will be making use of a simple HelloWorldServer application that will let us run in the background and connect from a client using telnet. Please take a look at the full overview of the application to learn how to create and build the HelloWorldServer application for this example. Unless you want to actually run the example, it will suffice to say that it can be run using the following Java command line.
|
Running HelloWorldServer in a Console |
||||
The Wrapper has now been set up. Let's start testing our configuration by running the Wrapper in a Windows Command Prompt. Please open a new Command Prompt and go into our %EXAMPLE_HOME% directory with cd command. The Wrapper and our wrapped application can now be started as follows:
Note that the location of the configuration file is relative to the location of the Wrapper binary. This is a little counter-intuitive, but it greatly increases the reliability of the Wrapper when launched using different methods. When the Wrapper starts, you should see something like this:
The banner at the top of the log is because we are using a temporary trial license. It points you to where you can get a long term trial license or purchase a license later.
The line that starts by "Command: " is a debug message, it shows the exact Java command line the Wrapper is executing to start the JVM.
It is very useful to resolve problems.
Once it is working, please comment out the
wrapper. If you have purchased a license, this is what you would see:
You can stop your application at any time by pressing CTRL-C. The Wrapper always keeps a record of why an application was stopped so you will see something like the following in your log.
|
Simulate a Crash |
|
Now, to see a very simple example of how the Wrapper is able to recover from a number of failures. Please start up the Wrapper again. Next bring up the Windows Task Manager. This can be done by pressing CTRL-ALT-DEL. View the Processes tab and locate the java.exe process. Select it and press End Process. This will tell Windows to immediately kill the Java process without giving it a chance to shutdown nicely. When the Java process dies, you will see something like the following in the Wrapper log. Notice how the Wrapper detects that the JVM died and launches a replacement within a few seconds.
The log file keeps track of how many times the JVM has been restarted. This is very useful to system administrators so they can easily see whether there have been problems simply by looking at the end of the file. |
Reference: Launching your application with the Wrapper |
[Windows]
[Linux / Unix]
[Common] |