Java itself is a very large program which approaches an operating system in its complexity. While it is usually quite stable, like any program it occasionally has problems like crashes, freezes, etc. When a Java application process freezes, it can be difficult to detect and recover from. Because the Java process itself still exists in memory, many external monitoring applications will think it is alive even though it has stopped responding.
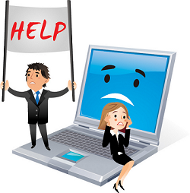
When a JVM freeze occurs, it is usually noticed by users or other systems failing to interact with the application. Once notified of the problem, often via a complaint from a user, a system administrator then must connect to the server, kill the frozen JVM, and restart the application. All of this can potentially take a couple hours if the person who needs to do the work is out at dinner.
Solution |
||
The solution to this problem is to have a monitoring application, with an intimate knowledge of how Java works, which can be trusted to monitor your Java application 24 hours a day, 365 days a year, and automatically take care of problems when they arise. The Java Service Wrapper contains several monitoring features. One of which is the ability to detect when a JVM has frozen. The Wrapper constantly monitors a JVM, and contains advanced logic to decide whether or not a JVM is frozen. When a frozen JVM is detected, the Wrapper is then able to automatically restart the JVM to get the system back up and running with a minimum of delay. The Wrapper can then send out an email notification to the system administrators in case they wish to double check to make sure everything is back up and working normally. The Java Service Wrapper periodically pings the JVM process (every 5 seconds by default) and waits for a response. If the response fails to arrive within a configured period of time (30 seconds by default), then the Wrapper will determine that it is frozen. The Wrapper also takes into account things like overall system load to make sure that false positives are kept to a minimum. When a freeze is detected, the Wrapper will record something like the following in the log file:
The Wrapper helps you detect critical problems including: |
Technical Overview |
||||||||||||||||||||||||||||||||||||||||||||
Freeze detection is enabled by default.
It will attempt to ping the JVM every 5 seconds
and then timeout after 30 seconds without a response.
Both of these intervals are configurable.
If you set the wrapper.
|
Reference: Freezes |
The Java Service Wrapper provides a full set of configuration properties that allows you to make the Wrapper meet your exact needs. Please take a look at the documentation for the individual properties to see all of the possibilities beyond the examples shown above.
|