Java as a language was designed to make it impossible for user developed code to result in an application crash . Any error would result in a nice clean exception being thrown which could be caught, and then handled appropriately. The reality, as any long time Java developer or system administrator knows, is that the Java Virtual Machine (JVM) itself can and does crash. This is because the JVM is itself a program written in native code, and like any very large complicated program, the JVM has some bugs in it.
Like any program, when Java crashes, it simply dies and goes away along with your application. This can be disastrous if the application is a mission critical system. The application will be down, and the outage may not be noticed until a customer visits your web site, or the shipping department calls because they haven't received any shipping orders for a few hours. Once the fact that the application is down is detected, it can potentially take an hour or two longer for the system administrator to come in and restart it.
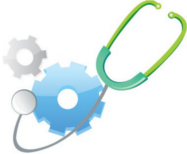
- Solution
- Technical Overview
- Trying it out
- Troubleshooting
- Restart Delay
- Disabling Restarts
- Responding to Events
- Email Notification
- Service Recovery
- Reference Properties
Solution |
||
The solution to this problem is to have a monitoring application, with an intimate knowledge about how Java works. It must be trusted to monitor your Java application 24 hours a day, 365 days a year, and automatically take care of problems when they arise. The Java Service Wrapper contains several monitoring features, one of which is the ability to detect when a JVM has crashed. It constantly monitors the JVM, and is able to instantly recognize when the JVM has crashed. As soon as a crash is detected, the Java Service Wrapper automatically restarts the JVM to get the system back up and running with a minimum of delay. Finally, you can configure an email notification to be send out to the system administrator to make sure everything is back up and working normally. The entire crash detection, recovery, and notification process is all handled by the Java Service Wrapper automatically without the need for a human operator to take action. When a crash is detected, the Java Service Wrapper will record something like the following in the log file:
While the above message may seem a little cryptic, it is the JVM's attempt to provide you with information about the crash. Because this output is dumped to the console at a very low level by the JVM, it is impossible for any Java based logging tools to record this information. Notice that the Wrapper is able to capture 100% of the console output of the JVM and make sure that it is all preserved in the log file. Without the Wrapper, not only would your application remain down, but the explanation of what happened would also be lost. Ideally, any crash should be fixed as soon as possible to avoid future crashes. However, because many crashes are caused by obscure interactions between perfectly valid Java code and bugs in the JVM itself, it can often take weeks or months for developers to identify and work around the problem. The Wrapper helps you detect critical problems including: |
Technical Overview |
||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||||
Crash detection in the Wrapper is always enabled as checking for crashes does not place any additional load on the JVM or system. As crashes either happen or they don't, there is no way to disable their detection. It is however possible to control what the Wrapper does when a crash does take place. When a crash is detected, the Wrapper will first wait a moment (5 seconds by default) to allow system memory and resources to settle back to normal, and then after any configured notifications, launch a new JVM instance to get your application back up and running without delay. As described below, it is possible to disable this auto restart functionality if needed.
|
Reference: Crashes |
The Java Service Wrapper provides a full set of configuration properties that allows you to make the Wrapper meet your exact needs. Please take a look at the documentation for the individual properties to see all of the possibilities beyond the examples shown above.
|